Hello to all,
The M5StickCPlus2 has a weird behaviour in regards to the I2c bus.
Before my M5Stick arrived, I tested the M5Stack Mini Scale with my Esp32 (Doit Devkit 1) successfully with the following code.
#include <Arduino.h>
#include <M5Unified.h>
#include "UNIT_SCALES.h"
#include <WiFi.h>
#include <PubSubClient.h>
#include <InfluxDbClient.h>
#include <InfluxDbCloud.h>
#define millis_between_readings 500
// WiFi
const char* ssid = ".....";
const char* password = "....";
#if defined(ESP32)
#include <WiFiMulti.h>
WiFiMulti wifiMulti;
#define DEVICE "ESP32"
#elif defined(ESP8266)
#include <ESP8266WiFiMulti.h>
ESP8266WiFiMulti wifiMulti;
#define DEVICE "ESP8266"
#endif
#define INFLUXDB_URL "....."
#define INFLUXDB_TOKEN "....."
#define INFLUXDB_ORG "...."
#define INFLUXDB_BUCKET "...."
// Time zone info
#define TZ_INFO "UTC2"
// Declare InfluxDB client instance with preconfigured InfluxCloud certificate
InfluxDBClient client(INFLUXDB_URL, INFLUXDB_ORG, INFLUXDB_BUCKET, INFLUXDB_TOKEN, InfluxDbCloud2CACert);
// Declare Data point
Point sensorP("ESP32_1");
UNIT_SCALES scales;
void setup()
{
M5.begin();
// Setup wifi
Serial.print("Connecting to WiFi: [");
Serial.print(ssid);
Serial.print("] password: [");
Serial.print(password);
Serial.print("]");
Serial.println();
Serial.print("Connecting to wifi");
WiFi.mode(WIFI_STA);
wifiMulti.addAP(ssid, password);
WiFi.disconnect();
delay(1);
while (wifiMulti.run() != WL_CONNECTED) {
Serial.println(WiFi.status());
Serial.print(" ");
delay(1000);
}
Serial.println("Connected to the Wi-Fi network");
// Accurate time is necessary for certificate validation and writing in batches
// We use the NTP servers in your area as provided by: https://www.pool.ntp.org/zone/
// Syncing progress and the time will be printed to Serial.
timeSync(TZ_INFO, "pool.ntp.org", "time.nis.gov");
// Check server connection
if (client.validateConnection()) {
Serial.print("Connected to InfluxDB: ");
Serial.println(client.getServerUrl());
} else {
Serial.print("InfluxDB connection failed: ");
Serial.println(client.getLastErrorMessage());
}
sensorP.addTag("device", DEVICE);
sensorP.addTag("sensorType", "HT711");
while (!scales.begin(&Wire, 21, 22, DEVICE_DEFAULT_ADDR))
{
Serial.println("scales connect error");
M5.Lcd.print("scales connect error");
delay(1000);
}
}
char *toCharArray(String str)
{
return &str[0];
}
void loop()
{
// Clear fields for reusing the point. Tags will remain the same as set above.
sensorP.clearFields();
// Delay between measurements.
sensorP.addField("weight", scales.getWeight());
sensorP.addField("gap", scales.getGapValue());
sensorP.addField("adc", scales.getRawADC());
// Write point
if (!client.writePoint(sensorP)) {
Serial.print("InfluxDB write failed: ");
Serial.println(client.getLastErrorMessage());
}
Serial.println("Pausing till next reading");
delay(millis_between_readings);
}
Here is a picture of the wiring I used to bridge the gap between the M5 connector and the Devkit 1. (Green: VIN, Gray: GND, Purple: D21, White D22).
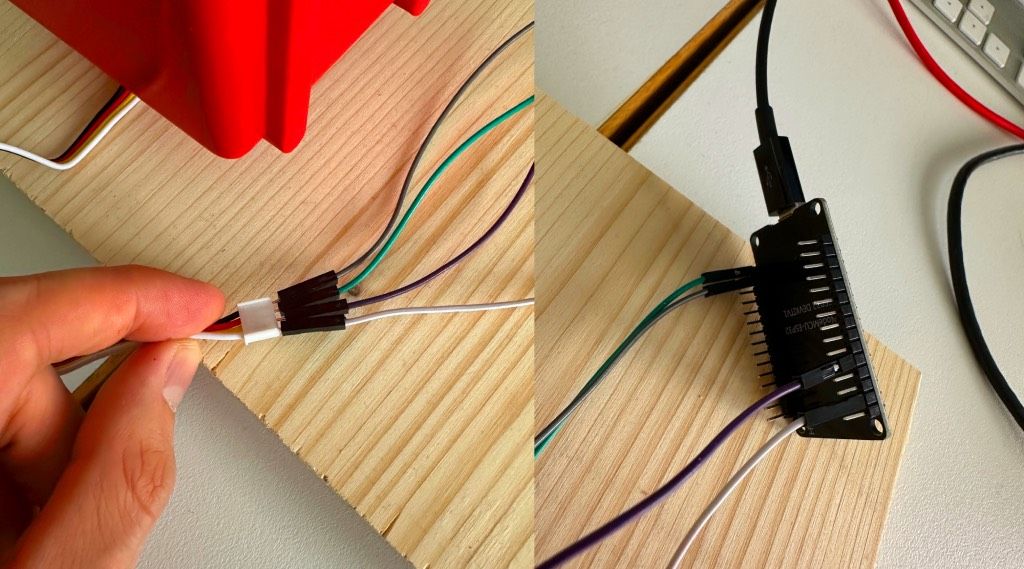
Anyone any idea what these two boards work differently?
Greetings Pascal